Preventing Updates to Specific Resources
To prevent specific resources in a stack from being modified by a stack update, you can create a stack policy when you create the stack. If you want to modify a stack policy or apply one to an existing stack, you can do so using the AWS CLI. You can’t remove a stack policy. A stack policy follows the same format as other resource policies and consists of the same Effect, Action, Principal, Resource, and Condition elements. The Effect functions the same as in other resource policies, but the Action, Principal, Resource, and Condition elements differ as follows:
Action The action must be one of the following:
Update:Modify Allows updates to the specific resource only if the update will modify and not replace or delete the resource
Update:Replace Allows updates to the specific resource only if the update will replace the resource
Update:Delete Allows updates to the specific resource only if the update will delete the resource
Update:* Allows all update actions
Principal The principal must always be the wildcard (). You can’t specify any other principal. Resource
This specifies the logical ID of the resource to which the policy applies. You must prefix this with the text LogicalResourceId/. Condition
This specifies the resource types, such as AWS::EC2::VPC. You can use wildcards to specify multiple resource types within a service, such as AWS::EC2:: to match all EC2 resources. If you use a wildcard, you must use the StringLike condition. Otherwise, you can use the StringEquals condition. The following stack policy document allows all stack updates except for those that would replace the PublicVPC resource. Such an update would include changing the VPC CIDR.
{
“Statement” : [
{
“Effect” : “Allow”,
“Action” : “Update:“, “Principal”: ““,
“Resource” : “*”
},
{ “Effect” : “Deny”,
“Action” : “Update:Replace”,
“Principal”: “*”,
“Resource” : “LogicalResourceId/PublicVPC”,
“Condition” : {
“StringLike” : {
“ResourceType” : [“AWS::EC2::VPC”]
}
}
}
]
}
CloudFormation doesn’t preemptively check whether an update will violate a stack policy. If you attempt to update a stack in such a way that’s prevented by the stack policy, CloudFormation will still attempt to update the stack. The update will fail only when CloudFormation attempts to perform an update prohibited by the stack policy. Therefore, when updating a stack, it’s important to verify that the update succeeded. Don’t just start the update and walk away.
Overriding Stack Policies
You can temporarily override a stack policy when doing a direct update. When you perform a direct update, you can specify a stack policy that overrides the existing one. CloudFormation will apply the updated policy during the update. Once the update is complete, CloudFormation will revert to the original policy.
CodeCommit
Git ( https://git-scm.com ) is a free and open-source version control system invented by Linus Torvalds to facilitate collaborative software development projects. However, people often use Git to store and track a variety of fi le-based assets, including source code, scripts, documents, and binary fi les. Git stores these fi les in a repository , colloquially referred to as a repo .
The AWS CodeCommit service hosts private Git-based repositories for version-controlled files, including code, documents, and binary files. CodeCommit provides advantages over other private Git repositories, including the following:
■ Encryption at rest using an AWS-managed key stored in KMS
■ Encryption in transit using HTTPS or SSH
■ No size limitation for repositories
■ 2 GB size limitation for a single file
■ Integration with other AWS services
A Git repository and S3 have some things in common. They both store files and provide automatic versioning, allowing you to revert to a previous version of a deleted or overwritten file. But Git tracks changes to individual files using a process called differencing, allowing you to see what changed in each file, who changed it, and when. It also allows you to create additional branches, which are essentially snapshots of an entire repository. For example, you could take an existing source code repository, create a new branch, and experiment on that branch without causing any problems in the original or master branch.
Creating a Repository
You create a repository in CodeCommit using the AWS management console or the AWS CLI. When you create a new repository in CodeCommit, it is always empty. You can add files to the repository in three ways.
■ Upload files using the CodeCommit management console.
■ Use Git to clone the empty repository locally, add files to it, commit those changes, and then push those changes to CodeCommit.
■ Use Git to push an existing local repository to CodeCommit.
Repository Security
CodeCommit uses IAM policies to control access to repositories. To help you control access to your repositories, AWS provides three managed policies. Each of the following policies allows access to all CodeCommit repositories using both the AWS Management Console and Git.
AWSCodeCommitFullAccess This policy provides unrestricted access to CodeCommit. This is the policy you’d generally assign to repository administrators.
AWSCodeCommitPowerUser This policy provides near full access to CodeCommit repositories but doesn’t allow the principal to delete repositories. This is the policy you’d assign to users who need both read and write access to repositories.
AWSCodeCommitReadOnly This grants read-only access to CodeCommit repositories. If you want to restrict access to a specific repository, you can copy one of these policies to your own customer-managed policy and specify the ARN of the repository.
Interacting with a Repository Using Git
Most users will interact with a CodeCommit repository via the Git command-line interface, which you can download from https://git-scm.com. Many integrated development environments (IDEs) such as Eclipse, IntelliJ, Visual Studio, and Xcode provide their own user-friendly Git-based tools. Only IAM principals can interact with a CodeCommit repository. AWS recommends generating a Git username and password for each IAM user from the IAM management console. You can generate up to two Git credentials per user. As CodeCommit doesn’t use resource-based policies, it doesn’t allow anonymous access to repositories. If you don’t want to configure IAM users or if you need to grant repository access to a federated user or application, you can grant repository access to a role. You can’t assign Git credentials to a role, so instead you must configure Git to use the AWS CLI Credential Helper to obtain temporary credentials that Git can use.
Git-based connections to CodeCommit must be encrypted in transit using either HTTPS or SSH. The easiest option is HTTPS, as it requires inputting only one’s Git credentials. However, if you can’t use Git credentials—perhaps because of security requirements—you can use SSH. If you go this route, you must generate public and private SSH keys for each IAM user and upload the public key to AWS. The user must also have permissions granted by the IAMUserSSHKeys AWS-managed policy.
Also read this topic: Introduction to Cloud Computing and AWS -1
CodeDeploy
CodeDeploy is a service that can deploy applications to EC2 or on-premises instances. You can use CodeDeploy to deploy binary executables, scripts, web assets, images, and anything else you can store in an S3 bucket or GitHub repository. You can also use CodeDeploy to deploy Lambda functions. CodeDeploy offers a number of advantages over manual deployments, including the following:
■ Simultaneous deployment to multiple instances or servers
■ Automatic rollback if there are deployment errors ■ Centralized monitoring and control of deployments
■ Deployment of multiple applications at once
The CodeDeploy Agent
The CodeDeploy agent is a service that runs on your Linux or Windows instances and performs the hands-on work of deploying the application onto an instance. You can install the CodeDeploy agent on an EC2 instance at launch using a Userdata script or you can bake it into an AMI. You can also use AWS Systems Manager to install it automatically. You’ll learn about Systems Manager later in the chapter.
Deployments
To deploy an application using CodeDeploy, you must create a deployment that defines the compute platform, which can be EC2/On-premises or Lambda, and the location of the application source files. CodeDeploy currently only supports deployments from S3 or GitHub. CodeDeploy doesn’t automatically perform deployments. If you want to automate deployments, you can use CodePipeline, which we’ll cover later in the chapter.
Deployment Groups
Prior to creating a deployment, you must create a deployment group to define which instances CodeDeploy will deploy your application to. A deployment group can be based on an EC2 Auto Scaling group, EC2 instance tags, or on-premises instance tags.
Deployment Types
When creating a deployment group for EC2 or on-premises instances, you must specify a deployment type. CodeDeploy gives you the following two different deployment types to give you control over how you deploy your applications.
In-Place Deployment
With an in-place deployment you deploy the application to existing instances. In-place deployments are useful for initial deployments to instances that don’t already have the application. These instances can be stand-alone or, in the case of EC2 instances, part of an existing Auto Scaling group. On each instance, the application is stopped and upgraded (if applicable) and then restarted. If the instance is behind an elastic load balancer, the instance is deregistered before the application is stopped and then reregistered to the load balancer after the deployment to the instance succeeds. Although an elastic load balancer isn’t required to perform an in-place deployment, having one already set up allows CodeDeploy to prevent traffic from going to instances that are in the middle of a deployment.
Blue/Green Deployment
A blue/green deployment is used to upgrade an existing application with minimal interruption. With Lambda deployments, CodeDeploy deploys a new version of a Lambda function and automatically shifts traffic to the new version. Lambda deployments always use the blue/green deployment type. In a blue/green deployment against EC2 instances, the existing instances in the deployment group are left untouched. A new set of instances is created to which CodeDeploy
deploys the application. Blue/green deployments require an existing Application, Classic, or Network Load balancer. CodeDeploy registers the instances to the load balancer’s target group after a successful deployment. At the same time, instances in the original environment are deregistered from the target group.
Note that if you’re using an Auto Scaling group, CodeDeploy will create a new Auto Scaling group with the same confi guration as the original. CodeDeploy will not modify the minimum, maximum, or desired capacity settings for an Auto Scaling group. You can choose to terminate the original instances or keep them running. You may choose to keep them running if you need to keep them available for testing or forensic analysis.
Deployment Configurations
When creating your deployment group, you must also select a deployment configuration . The deployment configuration defines the number of instances CodeDeploy simultaneously deploys to, as well as how many instances the deployment must succeed on for the entire deployment to be considered successful. The effect of a deployment configuration differs based on the deployment type. There are three preconfigured deployment configurations you can choose from: OneAtATime, HalfAtATime, and AllAtOnce.
OneAtATime
For both in-place and blue/green deployments, if the deployment group has more than one instance, CodeDeploy must successfully deploy the application to one instance before moving on to the next one. The overall deployment succeeds if the application is deployed successfully to all but the last instance. For example, if the deployment succeeds to the first two instances in a group of three, the entire deployment will succeed. If the deployment fails to any instance but the last one, the entire deployment fails. If the deployment group has only one instance, the overall deployment succeeds only if the deployment to the instance succeeds. For blue/green deployments, CodeDeploy reroutes traffic to each instance as deployment succeeds on the instance. If unable to reroute traffic to any instance except the last one, the entire deployment fails.
HalfAtATime
For in-place and blue/green deployments, CodeDeploy will deploy to up to half of the instances in the deployment group before moving on to the remaining instances. The entire deployment succeeds only if deployment to at least half of the instances succeeds. For blue/green deployments, CodeDeploy must be able to reroute traffic to at least half of the new instances for the entire deployment to succeed.
AllAtOnce
For in-place and blue/green deployments, CodeDeploy simultaneously deploys the application to as many instances as possible. If the application is deployed to at least one instance, the entire deployment succeeds. For blue/green deployments, the entire deployment succeeds if CodeDeploy reroutes traffic to at least one new instance.
People also ask this Questions
- What is a defense in depth security strategy how is it implemented?
- What is AWS Solution Architect?
- What is the role of AWS Solution Architect?
- Is AWS Solution Architect easy?
- What is AWS associate solutions architect?
- Is AWS Solutions Architect Associate exam hard?
Infocerts, 5B 306 Riverside Greens, Panvel, Raigad 410206 Maharashtra, India
Contact us – https://www.infocerts.com
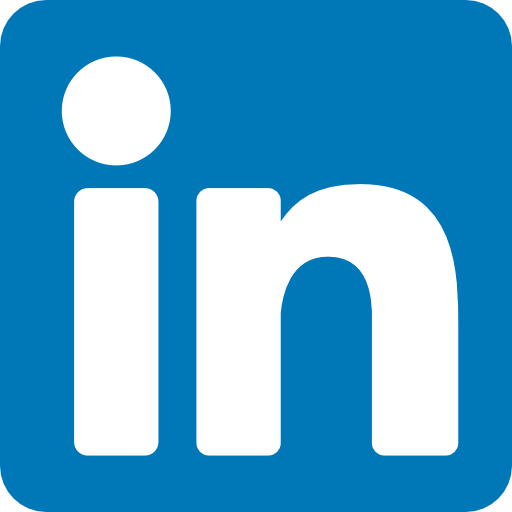